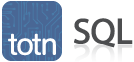
SQL: Practice Exercises for UPDATE Statement
If you want to test your skills using the SQL UPDATE statement, try some of our practice exercises.
These practice exercises allow you to test your skills with the UPDATE statement. You will be given questions that you need to solve. After each exercise, we provide the solution so you can check your answer.
Get started!
Practice Exercise #1:
Based on the products table populated with the following data, update the product_name to 'Grapefruit' for all records whose product_name is "Apple".
CREATE TABLE products ( product_id int NOT NULL, product_name char(50) NOT NULL, category_id int, CONSTRAINT products_pk PRIMARY KEY (product_id) ); INSERT INTO products (product_id, product_name, category_id) VALUES (1,'Pear',50); INSERT INTO products (product_id, product_name, category_id) VALUES (2,'Banana',50); INSERT INTO products (product_id, product_name, category_id) VALUES (3,'Orange',50); INSERT INTO products (product_id, product_name, category_id) VALUES (4,'Apple',50); INSERT INTO products (product_id, product_name, category_id) VALUES (5,'Bread',75); INSERT INTO products (product_id, product_name, category_id) VALUES (6,'Sliced Ham',25); INSERT INTO products (product_id, product_name, category_id) VALUES (7,'Kleenex',null);
Solution for Practice Exercise #1:
The following SQL UPDATE statement would perform this update.
Try ItUPDATE products SET product_name = 'Grapefruit' WHERE product_name = 'Apple';
The products table would now look like this:
product_id | product_name | category_id |
---|---|---|
1 | Pear | 50 |
2 | Banana | 50 |
3 | Orange | 50 |
4 | Grapefruit | 50 |
5 | Bread | 75 |
6 | Sliced Ham | 25 |
7 | Kleenex | NULL |
Practice Exercise #2:
Based on the suppliers table populated with the following data, update the city to 'Boise' and the state to "Idaho" for all records whose supplier_name is "Microsoft".
CREATE TABLE suppliers ( supplier_id int NOT NULL, supplier_name char(50) NOT NULL, city char(50), state char(50), CONSTRAINT suppliers_pk PRIMARY KEY (supplier_id) ); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (100, 'Microsoft', 'Redmond', 'Washington'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (200, 'Google', 'Mountain View', 'California'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (300, 'Oracle', 'Redwood City', 'California'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (400, 'Kimberly-Clark', 'Irving', 'Texas'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (500, 'Tyson Foods', 'Springdale', 'Arkansas'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (600, 'SC Johnson', 'Racine', 'Wisconsin'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (700, 'Dole Food Company', 'Westlake Village', 'California'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (800, 'Flowers Foods', 'Thomasville', 'Georgia'); INSERT INTO suppliers (supplier_id, supplier_name, city, state) VALUES (900, 'Electronic Arts', 'Redwood City', 'California');
Solution for Practice Exercise #2:
The following SQL UPDATE statement would perform this update in SQL.
Try ItUPDATE suppliers SET city = 'Boise', state = 'Idaho' WHERE supplier_name = 'Microsoft';
The suppliers table would now look like this:
supplier_id | supplier_name | city | state |
---|---|---|---|
100 | Microsoft | Boise | Idaho |
200 | Mountain View | California | |
300 | Oracle | Redwood City | California |
400 | Kimberly-Clark | Irving | Texas |
500 | Tyson Foods | Springdale | Arkansas |
600 | SC Johnson | Racine | Wisconsin |
700 | Dole Food Company | Westlake Village | California |
800 | Flowers Foods | Thomasville | Georgia |
900 | Electronic Arts | Redwood City | California |
Advertisements